Developers/Programs often have a need to interact with users, either to get data or to show some sort of result. Most programs use a dialog box as a way of asking the user to provide some type of input data. We have two inbuilt functions to read the input in Python from user.
- input ( prompt )
- raw_input ( prompt )
input (): This function takes the input from the user/keyboard and converts it into a string. The type of the returned object always will be a string <type ‘str’>. It does not evaluate/calculate the expression it just returns the complete statement as String. For example, Python have a built-in function called input() which takes the input from the user/keyboard. When the input() is called it stops the program and waits for the user’s input. When the user put-up input and presses enter, the program resumes and returns what the user typed.
Syntax:
inp = input('STATEMENT')
Example:
1. >>> name = input('What is your name?\n')
>>> What is your name?
Ajab
>>> print(name)
Ajab
# ---> comment in python
val
=
input
(
"Enter your value: "
)
print
(val)
name
=
input
(
'What is your name?\n'
)
print
(name)
How the input() function works in Python :
- When input() function start executes program flow will be paused until the user has given input.
- The message displayed on the output screen to ask a user to enter an input value is optional i.e. the prompt, which is optional.
- Whatever user enter as input, the input() function converts it into a string. if user enter an integer value still input() function converts it into a string. We can use typecasting to explicitly convert string to integer in our program.
num
=
input
(
"Enter number :"
)
print
(num)
name1
=
input
(
"Enter name : "
)
print
(name1)
print
(
"type of number"
,
type
(num))
print
(
"type of name"
,
type
(name1))
Taking input from console in Python
What is Console in Python? Console (also known as Shell) is basically a command line interpreter that takes input from the user/keyboard i.e one command at one time and interprets it. If there is no error then it runs the next command and gives required output otherwise display the error message. A Python Console looks like this.
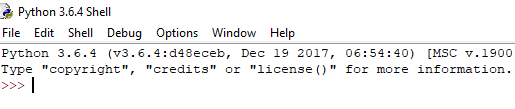
Here we write command and to run the command just press enter key and our command will be interpreted.
For coding in Python we must know the basics of the console used in
Python.
The primary shell of the python console is the three greater than symbols
We are free to write the next command on the prompt only when after
executing the first command.
The Python Console accepts command in Python which we write after the
prompt.
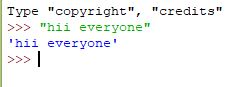
Accepting Input from Console
User enters the input in the Console and that input is then used in
the program as it was required.
To take input from the user we use a built-in function input().
This input is always stored as string value, we can also type cast this string value to integer, float or string by specifying the input() function inside the type.
- Typecasting the input to Integer:
- Typecasting the input to Float:
- Typecasting the input to String:
Taking multiple inputs from user in Python
The developer/Program often wants a user to enter multiple values or inputs in one line. In C++/C user can take multiple inputs in one line using scanf and cin>> respectively but in Python user can take multiple inputs in one line by two methods.
- Using split() method
- Using List comprehension
Using split() method :
This function helps us in getting multiple inputs from users. It breaks
the given values by the specified separator. If a separator is not
provided then any white space (white space) is considered as a separator.
Generally, users use a split method/function to split a Python string
but we can also use it in taking multiple inputs.
Syntax :
input().split(separator, maxsplit)
Example :
Output:
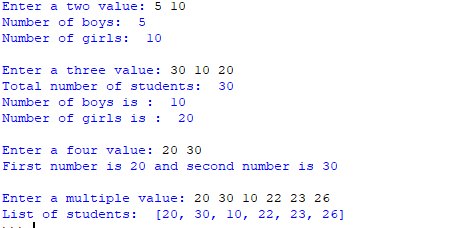
Using List comprehension :
List comprehension is an elegant way to create list in Python. We can
create lists just like mathematical statements in one line. It is also
used in getting multiple inputs from a user.
Output :
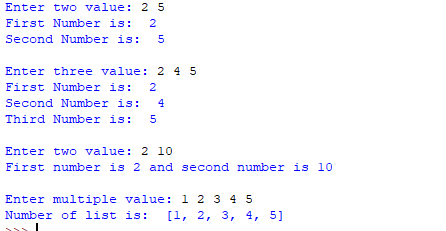
Python Input Methods for Competitive Programming
Python is an amazingly user-friendly programming language with the
only disadvantage of being slow. In comparison to C++, and Java, it
is quite slower. Online coding platforms, if C/C++ limit provided is
X. Usually, in Java time provided is 2X times and Python, it’s 5X times.
To improve the speed of code execution/running for input/output
intensive problems, languages have various input and output procedures.
An Example Problem :
Consider a question of finding the sum of N numbers inputted from the
user. Input a number N.
Input N numbers are separated by space in a line.
Examples:
Input :
5
1 2 3 4 5
Output :
15
0 Comments